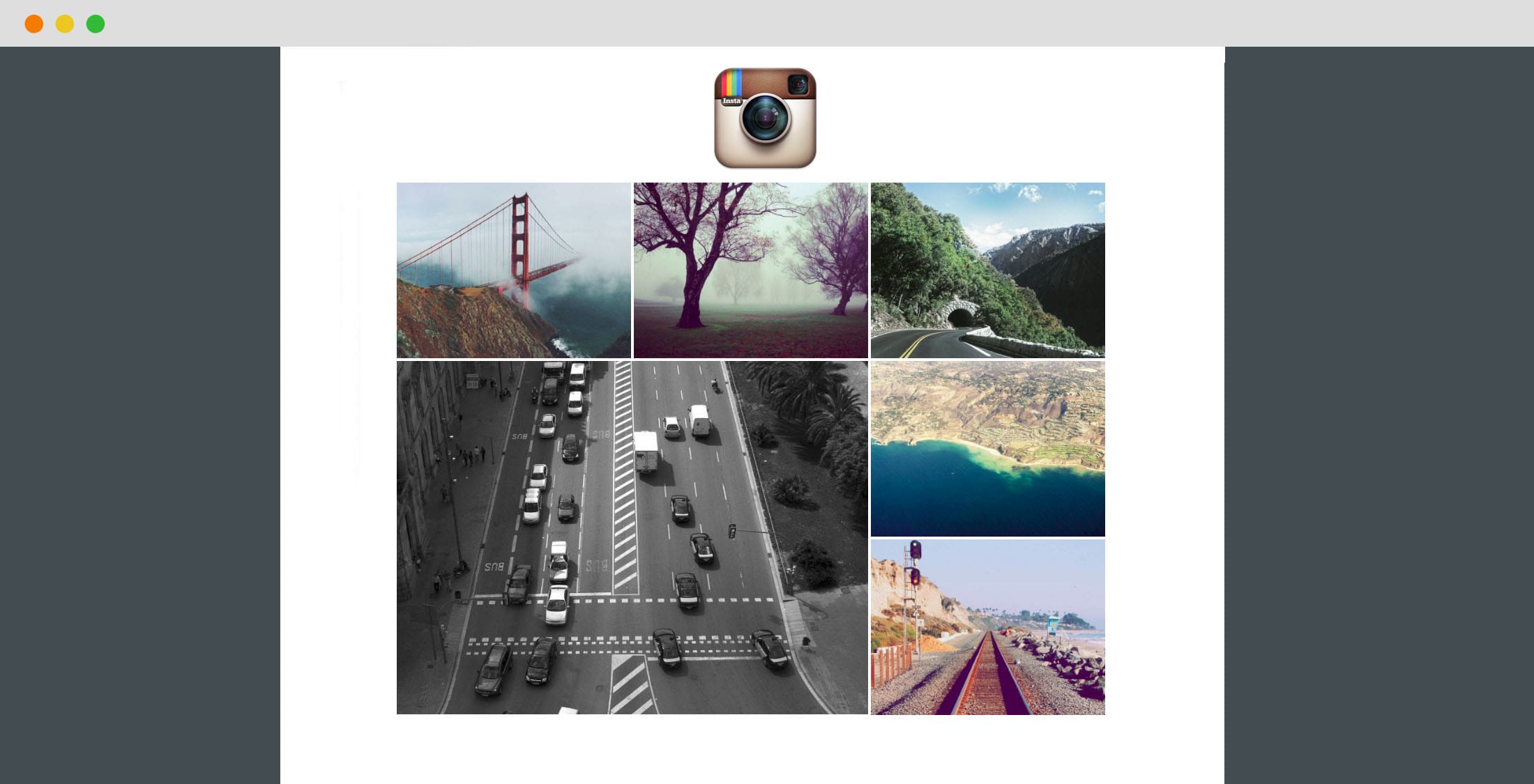
Instagram Feed – Building Gallery with Real-time Data via Instagram API
I recently wrote an article about building an RSS feed section to publish Facebook Instant Articles automatically. In this article, I will show how to build a gallery on your website with the picture you have uploaded to your Instagram account. In this tutorial I'll show you an easy way to pull down the data via API, using Vinkla’s Instagram package. I would like to thank him for creating a nice package.
API Credentials
Follow up the developer guide via their documentation, generate Client ID, Client Secret and you will need to go to another site to generate an access token.
Step 1
Let’s add new config array under existing file config/services.php
'instagram' => [
'access-token' => 'xxxxx.xxx.xxxx',
//replace xxx with your actual access token
],
Step 2
Adding a new route to handle the URL /instagram/feed
under routes/web.php
Route::get('/instagram/feed', [
'name' => 'Instagram Feed',
'as' => 'app.instagram.feed',
'uses' => 'InstagramController@feed',
]);
Step 3
Installing package via composer, use command from below or follow the installation guide from the package repository on github.
composer require vinkla/instagram php-http/message php-http/guzzle6-adapter
Step 4
Creating a new controller to handle the request and execute the API call.
<?php
namespace App\Http\Controllers;
use Vinkla\Instagram\Instagram;
/**
* Class InstagramController
* @package App\Http\Controllers
*/
class InstagramController extends Controller
{
/**
* @return mixed
*/
public function feed()
{
$instagram = new Instagram(config('services.instagram.access-token'));
$images = collect($instagram->get())->map(function ($each) {
return $each->images->standard_resolution->url;
});
return view('gallery', compact('images'));
}
}
Now, look at the code above in the controller, I have added the logic to pull down the Instagram feeds via API and utilized the Laravel collection map()
method to filter the images with standard resolution only. If you would like to show likes, a caption associated with those images you could customize that array and call them while rendering on the view file.
Step 5
Finally, its time to loop the images on gallery.blade.php file under resources/views.
<div class="tz-gallery">
<div class="row">
@foreach($images as $key => $image)
<div class="col-sm-12 col-md-4">
<a class="lightbox" href="{{ $image }}">
<img src="{{ $image }}" alt="Instagram Feed" class="img-responsive">
</a>
</div>
@endforeach
</div>
</div>
I have added a small block of code to just show the process of looping and displaying the images. The full source code I wrote for this tutorial is available on github, feel free to review the full pull request and utilize on your project.
Conclusion
Thanks for reading this article up to the end, if it was helpful to you, feel free share the knowledge with others, also don't forget to leave your feedback below in the comment section.
Happy Coding!